A gentle introduction to programming
This page includes examples in Python programming language. You
may want to try out some of the examples that come with the description.
To do this, execute the Python IDLE, which is available in the
Application-Menu of your operating system, and write the examples after
the prompt >>>
.
To get information about the IDLE, get access to the IDLE documentation
Writing any program consists of writing statements using a programming language. A statement is often known as a line of code that can be one of:
- comment,
- documentation,
- assignment,
- conditions,
- iterations, etc.
Lines of code are grouped in blocks. Depending on the programming language, blocks delimited by brackets, braces or by the indentation.
Each language has its own syntax to write these lines, and the user has to strictly follow this syntax for the program to be able to interpret the program. However, the amount of freedom the user has to use capital letters, whitespace and so on is very high. Recommendations for Python language are available in the PEP8 - Style Guide for Python Code.
Variables: Assignment and Typing
A variable is a name to give to a piece of memory with some information inside. Assignment is then the action of setting a variable to a value. The equal sign (=) is used to assign values to variables.
>>>a = 1 >>>b = 1.0 >>>c = "c" >>>hello = "Hello world!" >>>vrai = True
In the previous example, a
, b
,
c
, hello
and vrai
are variables,
a = 1
is a declaration.
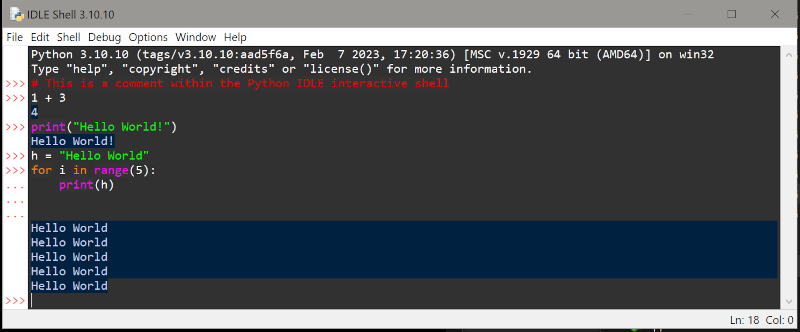
Assignments to variables with Python language can be performed with the following operators:
>>> a =
10 # simple assignment operator
>>> a += 2 # add and assignment operator, so 'a' is 12
>>> a -= 7 # minus and assignment, so 'a' is 5
>>> a *= 20 # multiply and assignment, so 'a' is 100
>>> a /= 10 # divide and assignment, so 'a' is 10
>>> a # verify the value of 'a'...
10
Basic Operators
Basic operators are used to manipulate variables. The following is the list of operators that can be used with Python, i.e. equal (assignment), plus, minus, multiply, divide:
Data types
The variables are of a data-type. For example, the declarations
a=1
and a=1.0
are respectively assigning an
integer and a real number. In Python, the command type
allows to get the variable type, like in the following:
>>> type(a)
<type 'int'>
>>> type(b)
<type 'float'>
>>> type(c)
<type 'str'>
>>> type(cc)
<type 'unicode'>
>>> type(vrai)
<type 'bool'>
Here is a list of some fundamental data types, and their characteristics:
- str String of characters
- unicode Unicode string of characters
- int Integer in range from -2147483648 to 2147483647
- bool Boolean value, can take value
True
(=1) orFalse
(=0) - float Floating point number (max 15 digits)
Python is assigning data types dynamically. As a consequence, the
result of the sum between an int
and a float
is a float
. The next examples illustrate that
the variable types have to be carefully managed.
>>> a =
10
>>> a += 0.
>>> a
10.0
>>> a += True
>>> a
11.0
>>> a += "a"
Traceback (most recent call
last):"<input>", line 1, in <module>
File TypeError: unsupported operand type(s) for +=: 'float' and 'str'
>>> a = "a"
>>> a *= 5
>>> a
'aaaaa'
The variable type can be explicitly changed. This is called a
cast
:
>>> a =
10
>>> b = 2
>>> a/b
5
>>> float(a) / float(b)
5.0
>>> a = 1
>>> b = 1
>>> str(a) + str(b)
'11'
Complex data types are often used to store variables sharing the same
properties, like, for example, a list of numbers. Common types in languages
are lists/arrays and dictionaries. The following is the assignment of a
list with name fruits
, then the assignment of a sub-part of
the list to the to_buy
list:
Conditions
Conditions aim to test whether a statement is True or False. The statement of the condition can include a variable, or be a variable and is written with operators. The following shows examples of conditions/comparisons in Python. Notice that comparing variables of a different data-type is possible but not recommended!
>>> var =
100
>>> if var == 100:
print("Value of expression is 100.")
...
...is 100.
Value of expression
>>> if var == "100":
print("This message won't be printed
out.")
... ...
Conditions can be expressed in a more complex way like:
>>> if a
== b:
print('a and b are equals')
... elif a > b:
... print('a is greater than b')
... else:
... print('b is greater than
a') ...
The simple operators for comparisons are summarized in the next examples:
>>> a == b
# check if equals
>>> a != b # check if
different
>>> a > b # check if 'a' is
greater than 'b'
>>> a >= b # check if 'a' is
greater or equal to 'b'
>>> a < b # check if 'a' is
lesser than 'b'
>>> a <= b # check if 'a' is
lesser or equal to 'b'
It is also possible to use the following operators:
and
: calledLogical AND operator
. If both the operands are 'True' then the condition becomes true.or
: calledLogical OR operator
. If any of the two operands are non-zero then the condition becomes 'True'.not
calledLogical NOT operator
. Use to reverse the logical state of its operand. If a condition is true then Logical NOT operator will make false.in
: evaluates to true if it finds a variable in the specified sequence and false otherwise.
Loops
The for
loop statement iterates over the items of any
sequence. The next Python lines print items of a list on the
screen:
>>> to_buy = ['fruits', 'viande', 'poisson', 'oeufs']
>>> for item in
to_buy:
print(item)
...
...
fruits
viande
poisson oeufs
A while
loop statement repeatedly executes a target
statement as long as a given condition returns True
. The
next example prints exactly the same result as the previous
one:
Dictionaries
A dictionary is a beneficial data type. It consists of pairs of keys and their corresponding values.
fruits['apples']
is a way to get the value - i.e., 3, of
the apple
key. However, an error is sent if the key is
unknown, like fruits[bananas]
. Alternatively, the
get
function can be used, like
fruits.get("bananas", 0)
that returns 0 instead of an
error.
The next example is showing how use a simple dictionary:
>>> for key
in fruits:
= fruits.get(key, 0)
... value if value < 3:
... print("You have to buy new {:s}.".format(key))
...
... You have to buy new
tomatoes.
To learn more about data structures and how to manage them, get access to the Python documentation